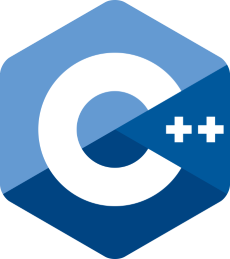
Now, for each pair of different waypoints, we:
This is the main part of the algorithm. Here, given a starting waypoint and an ending waypoint, the algorithm has to return a list of waypoints forming the shortest path (minimal cost according to constraints provided) from start to end.
From the starting point, we compute the cost of moving to the neighbors and keep those neighbors as candidates for the next move. We then select the candidate with lesser cost, compute the cost of moving to its non-visited neighbors and add them to the list of candidates, and so on until there are no more candidates to evaluate. Every time we update the cost of moving to a neighbor we keep track of the waypoint where we should move from. This, as it is, is the famous Dijkstra’s algorithm, the difference from the A* will be the addition of a heuristic when sampling the next waypoint candidate.
Here, I will compute the cost of moving from a waypoint A to a waypoint B as cost(A) + distance(A, B)
where cost(A)
returns the cost from the starting waypoint to waypoint A and distance()
returns the euclidian distance between two waypoints. However, in my example, when sampling a candidate I want to get one that has min(cost(candidate) + distance(candidate, endpoint))
, i.e., I will give preference to the one that has low cost and is close to the ending waypoint at same time. The heuristic allows us to obtain our result more quickly and it can be whatever you want or makes sense in your path finding, not necessarily distances.
The algorithm is something like:
It might be a bit confuse, I didn’t give my best to write that. The following gifs show the difference between A* (left) and Dijkstra (right), where the blue sphere is the ending waypoint, black is a visited waypoint, the range from yellow to red shows the cost (yellow -> low, red -> high) and green is the final path.
This code shows the preproces and path finding phases. You will find the full source code in the repository (link is in the end of this post). The code will probably change when I start adding more stuff in the repository, but I will try to keep this updated.
/// Check for viable paths and compute distances between waypoints.
void Preprocess()
{
if(m_Waypoints != null)
{
_Distances = new float[m_Waypoints.Length,m_Waypoints.Length];
// Clear all list of neighbors.
for(int waypoint1Id = 0; waypoint1Id < m_Waypoints.Length; ++waypoint1Id)
{
Waypoint waypoint1 = m_Waypoints[waypoint1Id];
waypoint1.m_Neighbors.Clear();
waypoint1.id = waypoint1Id;
}
for(int waypoint1Id = 0; waypoint1Id < m_Waypoints.Length; ++waypoint1Id)
{
Waypoint waypoint1 = m_Waypoints[waypoint1Id];
for(int waypoint2Id = waypoint1Id + 1; waypoint2Id < m_Waypoints.Length; ++waypoint2Id)
{
if(waypoint1Id != waypoint2Id)
{
Waypoint waypoint2 = m_Waypoints[waypoint2Id];
Vector3 direction = waypoint2.transform.position - waypoint1.transform.position;
float distance = direction.magnitude;
// If there is no obstacle between them, add each other to own neighborhood.
if(!Physics.Raycast(waypoint1.transform.position,direction.normalized, distance))
{
waypoint1.AddNeighbor(waypoint2);
waypoint2.AddNeighbor(waypoint1);
}
// Store distances.
_Distances[waypoint1Id, waypoint2Id] = distance;
_Distances[waypoint2Id, waypoint1Id] = distance;
}
}
}
}
}
// Store visited waypoints. Once the path is computed clear only the visited ones.
Queue<Waypoint> _VisitedWaypoints = new Queue<Waypoint>();
// list with waypoints and the cost of moving to it, sorted by cost.
SortedList<float, Waypoint> _SortedList = new SortedList<float, Waypoint>();
///
/// Compute a path from startWaypoint to endWaypoint using A-Star algorithm.
///
public Stack<Waypoint> GetPath(Waypoint startWaypoint, Waypoint endWaypoint)
{
Stack<Waypoint> path = new Stack<Waypoint>();
// If endWaypoint has a direct access from startWaypoint, nothing to do here.
if(startWaypoint.IsNeighbor(endWaypoint))
{
path.Push(endWaypoint);
path.Push(startWaypoint);
} else
{
_SortedList.Clear();
// Add startWaypoint with cost of 0.
startWaypoint.m_Cost = 0;
_SortedList.Add(0, startWaypoint);
_VisitedWaypoints.Enqueue(startWaypoint);
Waypoint currentWaypoint = null;
// continue while there are waypoints to visit AND the currentWaypoint is not the endWaypoint
while(_SortedList.Count > 0 && (currentWaypoint = _SortedList[_SortedList.Keys[0]]) != endWaypoint)
{
// Mark waypoint as visited and remove from list.
currentWaypoint.m_Visited = true;
_SortedList.RemoveAt(0);
for(int neighborId = 0; neighborId < currentWaypoint.m_Neighbors.Count; ++neighborId)
{
Waypoint neighborWaypoint = currentWaypoint.m_Neighbors[neighborId];
// Check not visited neighbors.
if(!neighborWaypoint.m_Visited)
{
// Is it cheaper to move from the current waypoint to this neighbor or the previous movement to this neighbor was better?
// ==
// The currentWaypoint cost + distance from currentWaypoint to this neighborWaypoint
// is lesser than the current cost of the neighborWaypoint?
float cost = currentWaypoint.m_Cost + _Distances[currentWaypoint.id, neighborWaypoint.id];
if(cost < neighborWaypoint.m_Cost)
{
// if so, keep the new value and say that is better move from currentWaypoint to neighborWaypoint instead.
neighborWaypoint.m_Cost = cost;
neighborWaypoint.m_Previous = currentWaypoint;
// Add neighborWaypoint to the list to be visited, but add to its cost the distance from it to the endWaypoint.
// This is the heuristic we are using for the A-star. This way, the closest ones to the end will be selected first.
_SortedList.Add(neighborWaypoint.m_Cost + _Distances[neighborWaypoint.id, endWaypoint.id], neighborWaypoint);
_VisitedWaypoints.Enqueue(neighborWaypoint);
}
}
}
}
// No path found.
if(currentWaypoint != endWaypoint)
{
return null;
}
// Track back the path, from end to start, and store in a stack (LIFO).
Waypoint waypoint = endWaypoint;
while(waypoint != null)
{
path.Push(waypoint);
waypoint = waypoint.m_Previous;
}
// Reset settings of visited points.
while(_VisitedWaypoints.Count > 0)
{
_VisitedWaypoints.Dequeue().Reset();
}
}
return path;
}
This is just a naive implementation to give a general idea about the A*. This is an old algorithm and several improvements have been made to it since its first appearance. Also, it would be impossible to describe a general solution, given that each game has its own demands and restrictions. The heuristics used has to be adapted accordingly. For example, one might add cost for slopes in terrain or for areas considered dangerous in the environment.
I created this new separate repository only for crowd simulation stuff. This A* implementation is already there.
The next post in this series will probably be about navigation meshes. Stay tuned.
Unity’s Scriptable Render Pipeline represents a great advance on the way that unity deals with graphics, giving more power to the users to customize the pipeline the way they want....
Read MoreContinuing the posts of stuff that I should have posted last year but for some reason didn’t. Here, some (not deep) thoughts on Cook-Torrance and lookup textures.
Read More